PythonでJSONファイルを扱ってみる。
標準ライブラリ json
json.load()
で読み込むjson.dump()
で書き出す
目次
標準ライブラリ json でJSONファイルを扱う
設定値を定義したJSONファイルを読み込んで使用するケースを想定します。
{
"app": "Sample App",
"version": "1.0.0",
"web": {
"host": "localhost",
"port": 8080
},
"db": {
"host": "localhost",
"port": 5432,
"authentication": {
"user": "admin",
"password": "PW#rew8bMV5"
}
},
"testUsers": [
"taro",
"jiro",
"saburo"
]
}
pip install json は不要
json は Python の標準ライブラリです。
import json
と書けばすぐに使えます。
ちなみに pip install すると、エラーが出ます。
$ pip install json
ERROR: Could not find a version that satisfies the requirement json (from versions: none)
ERROR: No matching distribution found for json
load で dict に変換
openでファイルを読み込み、json.load()
で 辞書 (dictionary)型に変換します。
辞書型なので、設定値を簡単に扱うことができます。
import json
if __name__ == '__main__':
with open('settings.json', 'r') as f:
settings = json.load(f)
# 型を取得
print(type(settings)) # <class 'dict'>
# app を取得
print(settings['app']) # Sample App
# dict.get() で取得
print(settings.get('web')) # {'host': 'localhost', 'port': 8080}
print(settings.get('testUsers')) # ['taro', 'jiro', 'saburo']
print(settings.get('nothing')) # None
# 深いネストの値を取得
print(settings['db']['authentication']['user']) # admin
dump でJSONファイルを書き出す
JSONファイルの値を元に、別のJSONファルに書き出してみます。
そのままでは面白くないので、値を書き換えた上で書き出します。
import json
if __name__ == '__main__':
with open('settings.json', 'r') as f:
settings = json.load(f)
# 値の書き換え
settings['db']['authentication']['user'] = 'pg_admin'
print(settings['db']['authentication']['user']) # pg_admin
# dump で書き出す
with open('settings_one_line.json', 'w') as f:
json.dump(settings, f)
with open('settings_indent.json', 'w') as f:
json.dump(settings, f, indent=4)
書き出したJSONファイルはそれぞれ、以下のようになります。
改行なし
改行がないため、見づらいです。
{"app": "Sample App", "version": "1.0.0", "web": {"host": "localhost", "port": 8080}, "db": {"host": "localhost", "port": 5432, "authentication": {"user": "pg_admin", "password": "PW#rew8bMV5"}}, "testUsers": ["taro", "jiro", "saburo"]}
改行あり・インデントあり(半角スペース 4つ)
見慣れた形式になりました。
人間が確認する場合はインデントありで書き出してあげた方が親切ですね ψ(・ω´・,,ψ
{
"app": "Sample App",
"version": "1.0.0",
"web": {
"host": "localhost",
"port": 8080
},
"db": {
"host": "localhost",
"port": 5432,
"authentication": {
"user": "pg_admin",
"password": "PW#rew8bMV5"
}
},
"testUsers": [
"taro",
"jiro",
"saburo"
]
}
まとめ
Pythonで簡単にJSONファイルを扱うことができました。
サクッと実行するプログラムを書くときなど、設定ファイルはJSONファイルで個別に管理するのも良さそうです。
参考
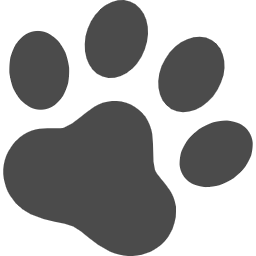
コメント